int TestID=rmCreateArea("Test ID Area");
rmSetAreaSize(TestID, 0.10, 0.10);
rmSetAreaLocation(TestID, .5, .5);
rmSetAreaWarnFailure(TestID, true);
rmSetAreaSmoothDistance(TestID, 50);
rmSetAreaCoherence(TestID, 1.0);
rmSetAreaMix(TestID, "himalayas_a");
rmSetAreaElevationType(TestID, cElevTurbulence);
rmSetAreaElevationVariation(TestID, 4.0);
rmSetAreaBaseHeight(TestID, 4.0);
rmSetAreaElevationOctaves(TestID, 3);
rmAddAreaInfluenceSegment(TestID, 1.0, 1.0, 0.0, 0.0);
rmSetAreaObeyWorldCircleConstraint(TestID, false);
rmBuildArea(TestID);
|
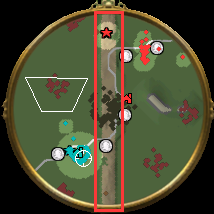 额外生成地形 |
int TestID=rmCreateArea("Test ID Area");
rmSetAreaSize(TestID, 0.05, 0.09);
rmSetAreaLocation(TestID, 0.3, 0.8);
rmSetAreaWarnFailure(TestID, true);
rmSetAreaSmoothDistance(TestID, 0);
rmSetAreaCoherence(TestID, 0.0);
rmSetAreaMix(TestID, "himalayas_a");
rmSetAreaElevationType(TestID, cElevTurbulence);
rmSetAreaElevationVariation(TestID, 4.0);
rmSetAreaBaseHeight(TestID, 4.0);
rmSetAreaElevationOctaves(TestID, 3);
rmSetAreaObeyWorldCircleConstraint(TestID, false);
rmBuildArea(TestID);
|
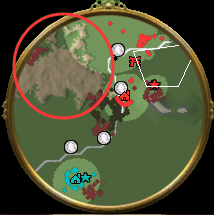 |
int TestID=rmCreateArea("Test ID Area");
rmSetAreaSize(TestID, 0.05, 0.09);
rmSetAreaLocation(TestID, 0.3, 0.8);
rmSetAreaWarnFailure(TestID, true);
rmSetAreaSmoothDistance(TestID, 50);
rmSetAreaCoherence(TestID, 0.0);
rmSetAreaMix(TestID, "himalayas_a");
rmSetAreaElevationType(TestID, cElevTurbulence);
rmSetAreaElevationVariation(TestID, 4.0);
rmSetAreaBaseHeight(TestID, 4.0);
rmSetAreaElevationOctaves(TestID, 3);
rmSetAreaObeyWorldCircleConstraint(TestID, false);
rmBuildArea(TestID);
|
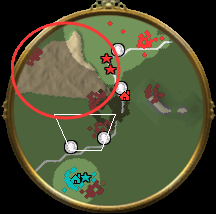 |
int TestID=rmCreateArea("Test ID Area");
rmSetAreaSize(TestID, 0.05, 0.09);
rmSetAreaLocation(TestID, 0.3, 0.8);
rmSetAreaWarnFailure(TestID, true);
rmSetAreaSmoothDistance(TestID, 50);
rmSetAreaCoherence(TestID, 1.0);
rmSetAreaMix(TestID, "himalayas_a");
rmSetAreaElevationType(TestID, cElevTurbulence);
rmSetAreaElevationVariation(TestID, 4.0);
rmSetAreaBaseHeight(TestID, 4.0);
rmSetAreaElevationOctaves(TestID, 3);
rmSetAreaObeyWorldCircleConstraint(TestID, false);
rmBuildArea(TestID);
|
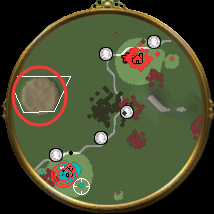 凝聚成圆形(范围0-1.0) |
int TestID=rmCreateArea("Test ID Area");
rmSetAreaSize(TestID, 0.05, 0.09);
rmSetAreaLocation(TestID, 0.3, 0.8);
rmSetAreaWarnFailure(TestID, true);
rmSetAreaSmoothDistance(TestID, 0);
rmSetAreaCoherence(TestID, 0.0);
rmSetAreaMix(TestID, "himalayas_a");
rmSetAreaElevationType(TestID, cElevTurbulence);
rmSetAreaElevationVariation(TestID, 4.0);
rmSetAreaBaseHeight(TestID, 4.0);
rmSetAreaObeyWorldCircleConstraint(TestID, false);
rmSetAreaBaseHeight(TestID, 10);
rmBuildArea(TestID);
|
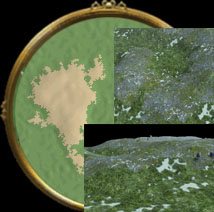 地形高度 |
int TestID=rmCreateArea("Test ID Area");
rmSetAreaSize(TestID, 0.05, 0.09);
rmSetAreaLocation(TestID, 0.3, 0.8);
rmSetAreaWarnFailure(TestID, true);
rmSetAreaSmoothDistance(TestID, 0);
rmSetAreaCoherence(TestID, 0.0);
rmSetAreaMix(TestID, "himalayas_a");
rmSetAreaElevationType(TestID, cElevTurbulence);
rmSetAreaElevationVariation(TestID, 4.0);
rmSetAreaBaseHeight(TestID, 4.0);
rmSetAreaObeyWorldCircleConstraint(TestID, false);
rmSetAreaBaseHeight(TestID, 10);
rmSetAreaHeightBlend(TestID, 100);
rmBuildArea(TestID);
|
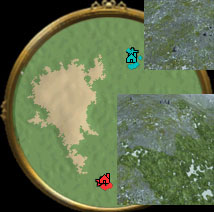 地形与周围地形平滑程度 |
int TestID=rmCreateArea("Test ID Area");
rmSetAreaSize(TestID, 0.05, 0.09);
rmSetAreaLocation(TestID, 0.3, 0.8);
rmSetAreaWarnFailure(TestID, true);
rmSetAreaSmoothDistance(TestID, 0);
rmSetAreaCoherence(TestID, 0.0);
rmSetAreaMix(TestID, "himalayas_a");
rmSetAreaElevationType(TestID, cElevTurbulence);
rmSetAreaElevationVariation(TestID, 4.0);
rmSetAreaBaseHeight(TestID, 4.0);
rmSetAreaObeyWorldCircleConstraint(TestID, false);
rmSetAreaBaseHeight(TestID, 10);
rmSetAreaHeightBlend(TestID, 100);
rmSetAreaReveal(TestID, -1);
rmBuildArea(TestID);
|
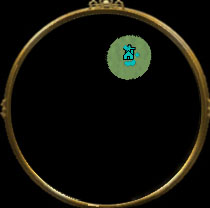 设定地形已探索:-1未探索 |
int TestID=rmCreateArea("Test ID Area");
rmSetAreaSize(TestID, 0.05, 0.09);
rmSetAreaLocation(TestID, 0.3, 0.8);
rmSetAreaWarnFailure(TestID, true);
rmSetAreaSmoothDistance(TestID, 0);
rmSetAreaCoherence(TestID, 0.0);
rmSetAreaMix(TestID, "himalayas_a");
rmSetAreaElevationType(TestID, cElevTurbulence);
rmSetAreaElevationVariation(TestID, 4.0);
rmSetAreaBaseHeight(TestID, 4.0);
rmSetAreaObeyWorldCircleConstraint(TestID, false);
rmSetAreaBaseHeight(TestID, 10);
rmSetAreaHeightBlend(TestID, 100);
rmSetAreaReveal(TestID, 0.0);
rmBuildArea(TestID);
|
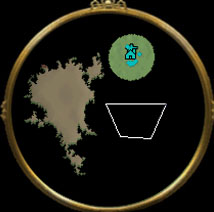 设定地形已探索:0已探索 |
int TestID=rmCreateArea("Test ID Area");
rmSetAreaSize(TestID, 0.05, 0.09);
rmSetAreaLocation(TestID, 0.3, 0.8);
rmSetAreaWarnFailure(TestID, true);
rmSetAreaSmoothDistance(TestID, 0);
rmSetAreaCoherence(TestID, 0.0);
rmSetAreaMix(TestID, "himalayas_a");
rmSetAreaElevationType(TestID, cElevTurbulence);
rmSetAreaElevationVariation(TestID, 4.0);
rmSetAreaBaseHeight(TestID, 4.0);
rmSetAreaObeyWorldCircleConstraint(TestID, false);
rmSetAreaBaseHeight(TestID, 10);
rmSetAreaHeightBlend(TestID, 100);
rmSetAreaReveal(TestID, 10.0);
rmBuildArea(TestID);
|
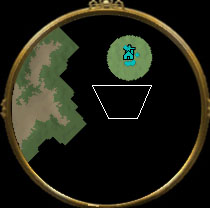 设定地形已探索:>1按距离 |
int TestID=rmCreateArea("Test ID Area");
rmSetAreaSize(TestID, 0.05, 0.09);
rmSetAreaLocation(TestID, 0.3, 0.8);
rmSetAreaWarnFailure(TestID, true);
rmSetAreaSmoothDistance(TestID, 0);
rmSetAreaCoherence(TestID, 0.0);
rmSetAreaMix(TestID, "himalayas_a");
rmSetAreaElevationType(TestID, cElevTurbulence);
rmSetAreaElevationVariation(TestID, 4.0);
rmSetAreaBaseHeight(TestID, 4.0);
rmSetAreaObeyWorldCircleConstraint(TestID, false);
rmSetAreaBaseHeight(TestID, 10);
rmSetAreaHeightBlend(TestID, 100);
rmSetAreaReveal(TestID, 100.0);
rmBuildArea(TestID);
|
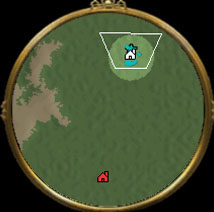 设定地形已探索:>1按距离 |
int TestID=rmCreateArea("Test ID Area");
rmSetAreaSize(TestID, 0.1, 0.1);
rmSetAreaLocation(TestID, 0.5, 0.5);
rmSetAreaWarnFailure(TestID, true);
rmSetAreaSmoothDistance(TestID, 0);
rmSetAreaCoherence(TestID, 0.5);
rmSetAreaMix(TestID, "himalayas_a");
rmSetAreaBaseHeight(TestID, 4.0);
rmSetAreaObeyWorldCircleConstraint(TestID, false);
rmSetAreaBaseHeight(TestID, 10);
rmSetAreaHeightBlend(TestID, 100);
rmSetAreaReveal(TestID, 0);
rmBuildArea(TestID);
|
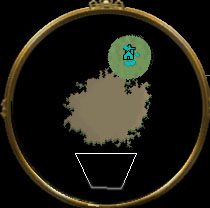 地形大小X0.1,Z0.1 凝聚性0.5 |
int TestID=rmCreateArea("Test ID Area");
rmSetAreaSize(TestID, 0.1, 0.3);
rmSetAreaLocation(TestID, 0.5, 0.5);
rmSetAreaWarnFailure(TestID, true);
rmSetAreaSmoothDistance(TestID, 0);
rmSetAreaCoherence(TestID, 0.5);
rmSetAreaMix(TestID, "himalayas_a");
rmSetAreaBaseHeight(TestID, 4.0);
rmSetAreaObeyWorldCircleConstraint(TestID, false);
rmSetAreaBaseHeight(TestID, 10);
rmSetAreaHeightBlend(TestID, 100);
rmSetAreaReveal(TestID, 0);
rmBuildArea(TestID);
|
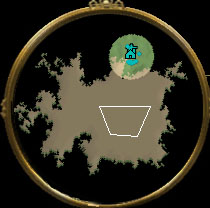 地形大小X0.1,Z0.3 凝聚性0.2 |
int TerrainSizeX=rmAreaTilesToFraction(100);
int TerrainSizeZ=rmAreaTilesToFraction(300);
int TestID=rmCreateArea("Test ID Area");
rmSetAreaSize(TestID, TerrainSizeX, TerrainSizeZ);
rmSetAreaLocation(TestID, 0.5, 0.5);
rmSetAreaWarnFailure(TestID, true);
rmSetAreaSmoothDistance(TestID, 0);
rmSetAreaCoherence(TestID, 0.2);
rmSetAreaMix(TestID, "himalayas_a");
rmSetAreaBaseHeight(TestID, 4.0);
rmSetAreaObeyWorldCircleConstraint(TestID, false);
rmSetAreaBaseHeight(TestID, 10);
rmSetAreaHeightBlend(TestID, 100);
rmSetAreaReveal(TestID, 0);
rmBuildArea(TestID);
|
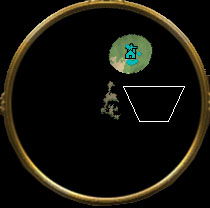 地形大小 X:rmAreaTilesToFraction(100) Z:rmAreaTilesToFraction(300) 凝聚性0.2 |
int TerrainSizeX=rmAreaTilesToFraction(1000);
int TerrainSizeZ=rmAreaTilesToFraction(3000);
int TestID=rmCreateArea("Test ID Area");
rmSetAreaSize(TestID, TerrainSizeX, TerrainSizeZ);
rmSetAreaLocation(TestID, 0.5, 0.5);
rmSetAreaWarnFailure(TestID, true);
rmSetAreaSmoothDistance(TestID, 0);
rmSetAreaCoherence(TestID, 0.2);
rmSetAreaMix(TestID, "himalayas_a");
rmSetAreaBaseHeight(TestID, 4.0);
rmSetAreaObeyWorldCircleConstraint(TestID, false);
rmSetAreaBaseHeight(TestID, 10);
rmSetAreaHeightBlend(TestID, 100);
rmSetAreaReveal(TestID, 0);
rmBuildArea(TestID);
|
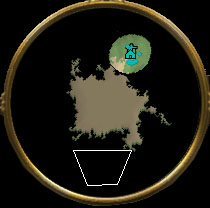 地形大小 X:rmAreaTilesToFraction(1000) Z:rmAreaTilesToFraction(3000) 凝聚性0.2 |
|
Debug.xml - 记事本 |
____ |
□ |
X |
|
|
文件(F) 编辑(E) 格式(O) 查看(V) 帮助(H) |
|
|
|
|
|
include "mercenaries.xs";
include "ypAsianInclude.xs";
include "ypKOTHInclude.xs";
void main(void)
{
// ---------------------------------------- Map Info -------------------------------------------
int playerTilesX=13200; //设定地图X大小
int playerTilesZ=13200; //设定地图Z大小(帝国3的Y是高度,Z才是我们平常所用到的Y)
//如果玩家大于4将playerTilesX与playerTilesZ改为11500(同一个值的int只能出现1次,当你需要修改数值的时候,不能再加入int)
if (cNumberNonGaiaPlayers >4){ playerTilesX=11500; playerTilesZ=11500;}
if (cNumberNonGaiaPlayers >6){ playerTilesX=10500; playerTilesZ=10500;} //Modify Map X&Z Size of 6,7,8 Player
int SizeX = 2*sqrt(cNumberNonGaiaPlayers*playerTilesX);
int SizeZ = 2*sqrt(cNumberNonGaiaPlayers*playerTilesZ);
string MapTerrain ="Carolinas\ground_marsh3_car"; //<-------- 地图地形,自己参照剧情编辑器 <--------
string MapLighting ="319a_Snow"; //<-------- 照明,自己参照剧情编辑器 <--------
string PlayerTerrain ="Carolinas\ground_marsh1_car"; //<--------- 玩家范围地形 <---------
//设定地图XZ大小,分别调用上面用int定义的SizeX与SizeZ,即为rmSetMapSize(13200,13200);如果玩家大于4将改为11500
rmSetMapSize(SizeX, SizeZ);
rmSetMapElevationParameters(cElevTurbulence, 0.15, 2.5, 0.35, 3.0); // type, frequency, octaves, persistence, variation
rmSetMapElevationHeightBlend(1.0);
//地形初始化,设定地图初始地形,调用上面用string定义MapTerrain,即为"Carolinas\ground_marsh3_car";
rmTerrainInitialize(MapTerrain,6);
//设定照明,调用上面用string定义MapLighting,即为"319a_Snow"
rmSetLightingSet(MapLighting);
rmSetGlobalRain(0.9); //设定下雨
chooseMercs();
rmSetMapType("yucatan");
rmSetMapType("water");
rmSetMapType("default"); //设定地图类型,地图类型影响到宝藏
rmSetMapType("land");
rmSetMapType("bayou");
rmSetSeaLevel(0); // this is height of river surface compared to surrounding land. River depth is in the river XML.
rmSetStatusText("",0.01);//读取地图进度条
rmPlacePlayersCircular(0.35, 0.35, 0.0); //圆形放置玩家
/*
int River1ID = rmRiverCreate(-1, "Northwest Territory Water", 1, 1, 10, 10);
rmRiverAddWaypoint(River1ID, 0.0, 0.2 );
rmRiverAddWaypoint(River1ID, 1.0, 0.2 );
rmRiverSetBankNoiseParams(River1ID,0.00, 0, 0.0, 0.0, 0.0, 0.0);
// rmRiverSetBankNoiseParams(River1ID,0.00, 0, 5.0, 5.0, 5.0, 5.0);
rmRiverAddShallows(River1ID,3,16);
rmRiverReveal(River1ID,0);
rmRiverBuild(River1ID);
int River2ID = rmRiverCreate(-1, "Northwest Territory Water", 1, 1, 10, 10);
rmRiverAddWaypoint(River2ID, 0.0, 0.8 );
rmRiverAddWaypoint(River2ID, 1.0, 0.8 );
rmRiverSetBankNoiseParams(River2ID,0.00, 0, 0.0, 0.0, 0.0, 0.0);
// rmRiverSetBankNoiseParams(River2ID,0.00, 0, 5.0, 5.0, 5.0, 5.0);
rmRiverSetShallowRadius(River2ID,16);
rmRiverAddShallow(River2ID,0.20);
rmRiverAddShallow(River2ID,0.50);
rmRiverAddShallow(River2ID,0.80);
rmSetAreaReveal(River2ID, 0);
rmRiverBuild(River2ID);
rmSetOceanReveal(true);
*/
//玩家范围
float AreaSizePlayer = rmAreaTilesToFraction(700);
for(i=1; <=cNumberNonGaiaPlayers)
{
int id=rmCreateArea("Player"+i);
rmSetPlayerArea(i, id);
rmSetAreaWarnFailure(id, false);
rmSetAreaSize(id, AreaSizePlayer, AreaSizePlayer);
rmSetAreaLocPlayer(id, i);
rmSetAreaCoherence(id, 0.85);
rmSetAreaSmoothDistance(id, 2);
rmSetAreaMinBlobs(id, 1);
rmSetAreaMaxBlobs(id, 1);
rmSetAreaTerrainType(id,PlayerTerrain);
rmBuildArea(id);
}
//定义城镇中心
int TownCenterID = rmCreateObjectDef("player TC");
if (rmGetNomadStart())
{
rmAddObjectDefItem(TownCenterID, "CoveredWagon", 1, 0.0);
}
else
{
rmAddObjectDefItem(TownCenterID, "TownCenter", 1, 0);
}
rmSetObjectDefMinDistance(TownCenterID, 0.0);
rmSetObjectDefMaxDistance(TownCenterID, 20.0);
for(i=1; <=cNumberNonGaiaPlayers)
{
rmPlaceObjectDefAtLoc(TownCenterID, i, rmPlayerLocXFraction(i), rmPlayerLocZFraction(i));
}
//定义起始单位(civs.xml定义那些开局单位)
int startingUnits = rmCreateStartingUnitsObjectDef(5.0);
rmSetObjectDefMinDistance(startingUnits, 6.0);
rmSetObjectDefMaxDistance(startingUnits, 10.0);
for(i=1; <=cNumberNonGaiaPlayers)
{
vector TCLocation = rmGetUnitPosition(rmGetUnitPlacedOfPlayer(TownCenterID, i));
rmPlaceObjectDefAtLoc(startingUnits, i, rmXMetersToFraction(xsVectorGetX(TCLocation)), rmZMetersToFraction(xsVectorGetZ(TCLocation)));
}
//这是避开边缘的写法。在0.5,0.5中心点开始算起,最小间隔距离为rmXFractionToMeters(0.0),最大间隔距离为rmXFractionToMeters(0.45)。
int avoidEdge = rmCreatePieConstraint("Avoid Edge",0.5,0.5, rmXFractionToMeters(0.0),rmXFractionToMeters(0.45), rmDegreesToRadians(0),rmDegreesToRadians(360));
//设定与城镇中心最小间隔25
int avoidTownCenter = rmCreateTypeDistanceConstraint("avoid Town Center", "townCenter", 25.0);
int TestID=rmCreateArea("Test ID Area");
rmSetAreaSize(TestID, rmAreaTilesToFraction(300), rmAreaTilesToFraction(300));
rmSetAreaWarnFailure(TestID, true);
rmSetAreaSmoothDistance(TestID, 0);
rmSetAreaCoherence(TestID, 0.2);
rmSetAreaMix(TestID, "himalayas_a");
rmSetAreaBaseHeight(TestID, 4.0);
rmSetAreaObeyWorldCircleConstraint(TestID, false);
rmSetAreaBaseHeight(TestID, 10);
rmSetAreaHeightBlend(TestID, 100);
rmSetAreaReveal(TestID, 0);
rmAddAreaConstraint(TestID, avoidTownCenter);
rmAddAreaConstraint(TestID, avoidEdge);
rmBuildArea(TestID);
//设定与TestID最小间隔25
int avoidArea1IDFar = rmCreateAreaDistanceConstraint("avoid AreaID1 Far", TestID, 25);
int Test2ID=rmCreateArea("Test ID Area2");
rmSetAreaSize(Test2ID, rmAreaTilesToFraction(300), rmAreaTilesToFraction(300));
rmSetAreaWarnFailure(Test2ID, true);
rmSetAreaSmoothDistance(Test2ID, 0);
rmSetAreaCoherence(Test2ID, 0.2);
rmSetAreaTerrainType(Test2ID, "Amazon\cliff_top1_ama");
rmSetAreaBaseHeight(Test2ID, 4.0);
rmSetAreaObeyWorldCircleConstraint(Test2ID, false);
rmSetAreaBaseHeight(Test2ID, 10);
rmSetAreaHeightBlend(Test2ID, 100);
rmSetAreaReveal(Test2ID, 0);
rmAddAreaConstraint(Test2ID, avoidArea1IDFar);
rmAddAreaConstraint(Test2ID, avoidTownCenter);
rmAddAreaConstraint(Test2ID, avoidEdge);
rmBuildArea(Test2ID);
rmSetStatusText("",0.50);//读取地图进度条
rmSetStatusText("",1.00);//读取地图进度条
//愿神兽会保佑你不会出错。
/*----------------------------------------------------------------------------------------------*
* ┏┓ ┏┓ *
* ┏┛┻━━━┛┻┓ *
* ┃ ┃ *
* ┃ ━ ┃ *
* ┃ ┳┛ ┗┳ ┃ *
* ┃ ┃ *
* ┃ ┻ ┃ *
* ┃ ┃ *
* ┗━┓ ┏━┛ Code is far away from bug with the animal protecting *
* ┃ ┃ *
* ┃ ┃ *
* ┃ ┃ *
* ┃ ┃ *
* ┃ ┃ *
* ┃ ┗━━━┓ *
* ┃ ┣┓ *
* ┃ ┏┛ *
* ┗┓┓┏━┳┓┏┛ *
* ┃┫┫ ┃┫┫ *
* ┗┻┛ ┗┻┛ *
*----------------------------------------------------------------------------------------------*/
} //END |
|
|
rmSetAreaTerrainType(int areaID, string terrainTypeName): Sets the terrain type for an area. |
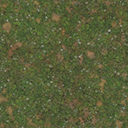 |
Amazon\cliff_top1_ama |
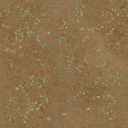 |
Amazon\ground_road_spc_ama |
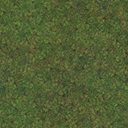 |
Amazon\ground1_ama |
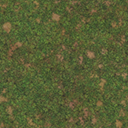 |
Amazon\ground2_ama |
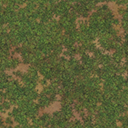 |
Amazon\ground3_ama |
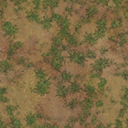 |
Amazon\ground4_ama |
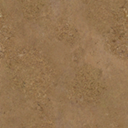 |
Amazon\ground5_ama |
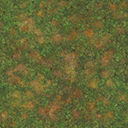 |
Amazon\groundforest_ama |
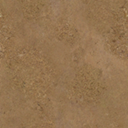 |
Amazon\river1_am |
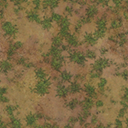 |
Amazon\river2_am |
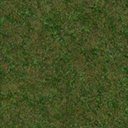 |
Amazon\river3_am |
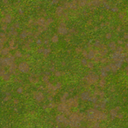 |
andes\ground07_and |
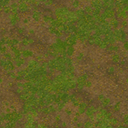 |
andes\ground08_and |
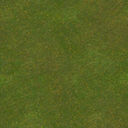 |
andes\ground09_and |
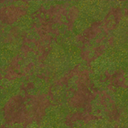 |
andes\ground10_and |
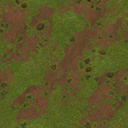 |
andes\ground11_and |
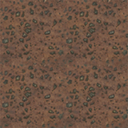 |
andes\ground12_and |
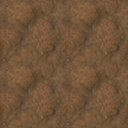 |
andes\ground14_and |
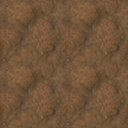 |
andes\ground14_and |
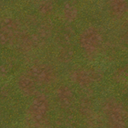 |
andes\ground16_and |
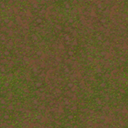 |
andes\ground17_and |
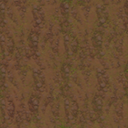 |
andes\ground18_and |
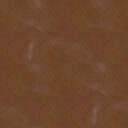 |
andes\ground22_and |
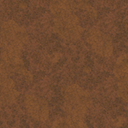 |
andes\ground25_and |
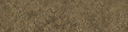 |
araucania\cliff_coast_ara |
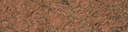 |
araucania\cliff_coast2_ara |
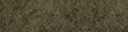 |
araucania\cliff_coast3_ara |
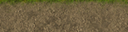 |
araucania\cliff_dirt_ara |
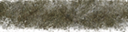 |
araucania\cliff_dirt_snow_ara |
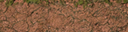 |
araucania\cliff_dirt2_ara |
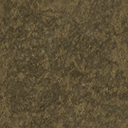 |
araucania\ground_dirt1_ara |
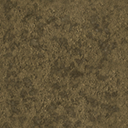 |
araucania\ground_dirt2_ara |
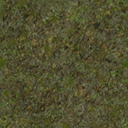 |
araucania\ground_dirt3_ara |
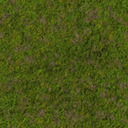 |
araucania\ground_grass1_ara |
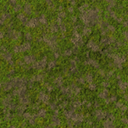 |
araucania\ground_grass2_ara |
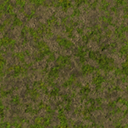 |
araucania\ground_grass3_ara |
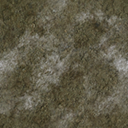 |
araucania\ground_snow1_ara |
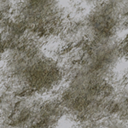 |
araucania\ground_snow2_ara |
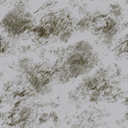 |
araucania\ground_snow3_ara |
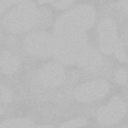 |
araucania\ground_snow4_ara |
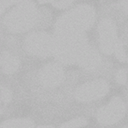 |
araucania\ground_snow5_ara |
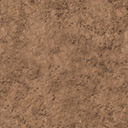 |
araucania\ground07_ara |
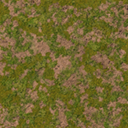 |
araucania\ground08_ara |
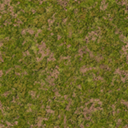 |
araucania\ground09_ara |
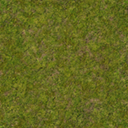 |
araucania\ground10_ara |
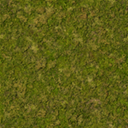 |
araucania\ground11_ara |
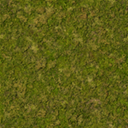 |
araucania\ground12_ara |
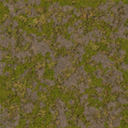 |
araucania\groundforestcentral |
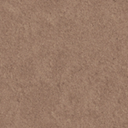 |
araucania\groundshore1_ara |
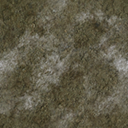 |
araucania\groundshore5_ara |
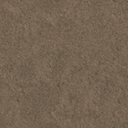 |
araucania\groundshore8_ara |
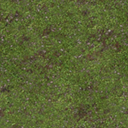 |
bayou\cliff_top1_bay |
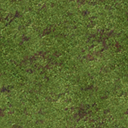 |
bayou\ground1_bay |
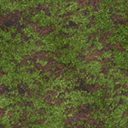 |
bayou\ground2_bay |
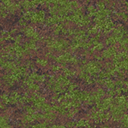 |
bayou\ground3_bay |
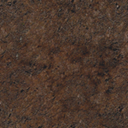 |
bayou\ground4_bay |
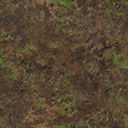 |
bayou\ground5_bay |
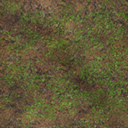 |
bayou\ground6_bay |
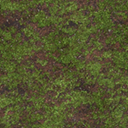 |
bayou\ground7_bay |
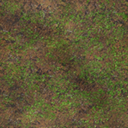 |
bayou\groundforest_bay |
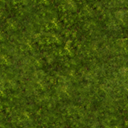 |
borneo\ground_forest_borneo |
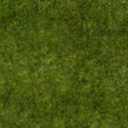 |
borneo\ground_grass1_borneo |
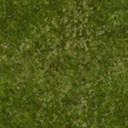 |
borneo\ground_grass2_borneo |
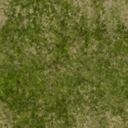 |
borneo\ground_grass3_borneo |
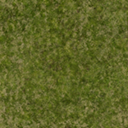 |
borneo\ground_grass4_borneo |
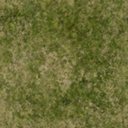 |
borneo\ground_grass5_borneo |
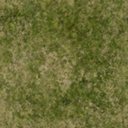 |
borneo\ground_grass5_borneo |
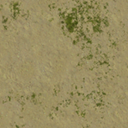 |
borneo\ground_sand1_borneo |
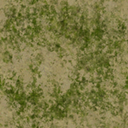 |
borneo\ground_sand2_borneo |
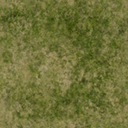 |
borneo\ground_sand3_borneo |
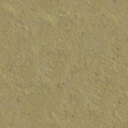 |
borneo\seafloor1_borneo |
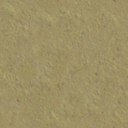 |
borneo\shoreline1_borneo |
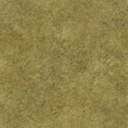 |
california\desert2_cal |
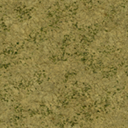 |
california\desert3_cal |
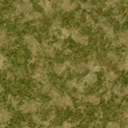 |
california\desert4_cal |
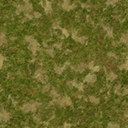 |
california\desert5_cal |
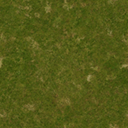 |
california\desert6_cal |
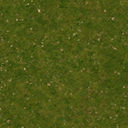 |
california\fakecalifgrassmix_cal |
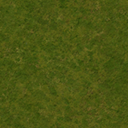 |
california\fakecalifgrassmix2_cal |
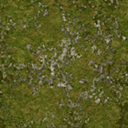 |
california\ground_clifftop_cal |
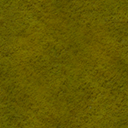 |
california\ground5_cal |
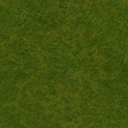 |
california\ground6_cal |
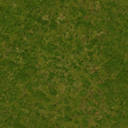 |
california\ground7_cal |
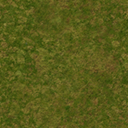 |
california\ground8_cal |
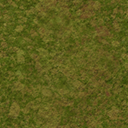 |
california\ground9_cal |
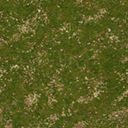 |
california\groundforest_cal |
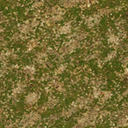 |
california\groundforest2_cal |
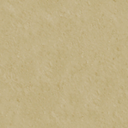 |
california\groundshore1_cal |
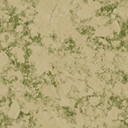 |
california\groundshore2_cal |
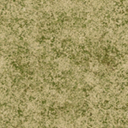 |
california\groundshore3b_cal |
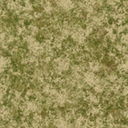 |
california\groundshore3c_cal |
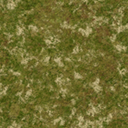 |
california\groundshore3d_cal |
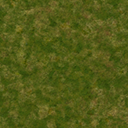 |
california\groundshore4_cal |
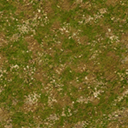 |
california\madrone_forest_cal |
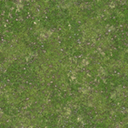 |
caribbean\cliff_top1_crb |
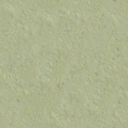 |
caribbean\ground_shoreline1_crb |
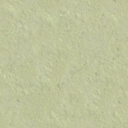 |
caribbean\ground_shoreline2_crb |
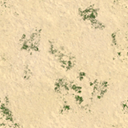 |
caribbean\ground_shoreline3_crb |
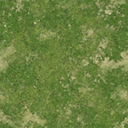 |
caribbean\ground1_crb |
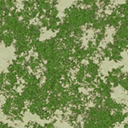 |
caribbean\ground2_crb |
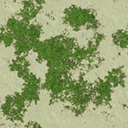 |
caribbean\ground3_crb |
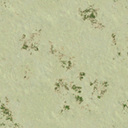 |
caribbean\ground4_crb |
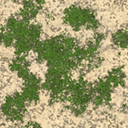 |
caribbean\ground5_crb |
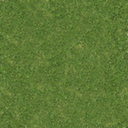 |
caribbean\ground6_crb |
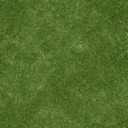 |
caribbean\ground7_crb |
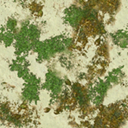 |
caribbean\groundforest_crb |
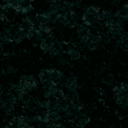 |
caribbean\seafloor_coral0_crb |
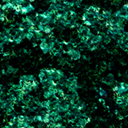 |
caribbean\seafloor_coral1_crb |
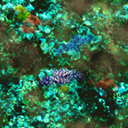 |
caribbean\seafloor_coral2_crb |
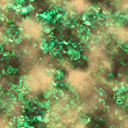 |
caribbean\seafloor_coral3_crb |
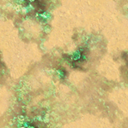 |
caribbean\seafloor_coral4_crb |
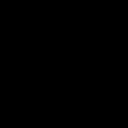 |
Carolinas\blackmap |
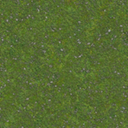 |
carolinas\cliff_top1_car |
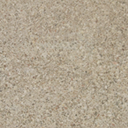 |
Carolinas\cobblestone |
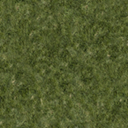 |
carolinas\ground_grass1_car |
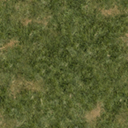 |
carolinas\ground_grass2_car |
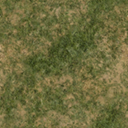 |
carolinas\ground_grass3_car |
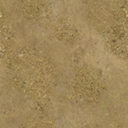 |
carolinas\ground_grass4_car |
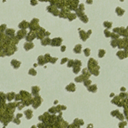 |
carolinas\ground_marsh1_car |
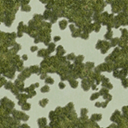 |
carolinas\ground_marsh2_car |
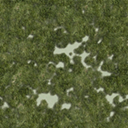 |
carolinas\ground_marsh3_car |
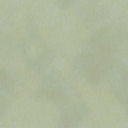 |
carolinas\ground_shoreline1_car |
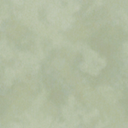 |
carolinas\ground_shoreline2_car |
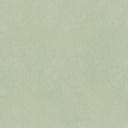 |
carolinas\ground_shoreline3_car |
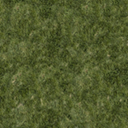 |
carolinas\groundforest_car |
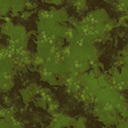 |
carolinas\groundforestmarsh_car |
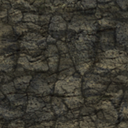 |
cave\cave_ground1 |
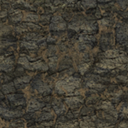 |
cave\cave_ground2 |
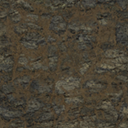 |
cave\cave_ground3 |
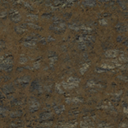 |
cave\cave_ground4 |
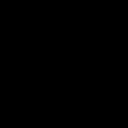 |
cave\cave_top |
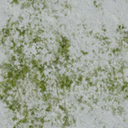 |
ceylon\clifftop_ceylon |
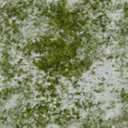 |
ceylon\ground_grass1_ceylon |
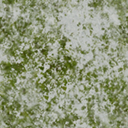 |
ceylon\ground_grass2_ceylon |
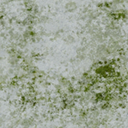 |
ceylon\ground_grass3_ceylon |
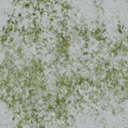 |
ceylon\ground_grass4_ceylon |
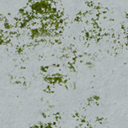 |
ceylon\ground_sand1_ceylon |
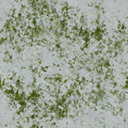 |
ceylon\ground_sand2_ceylon |
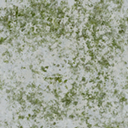 |
ceylon\ground_sand3_ceylon |
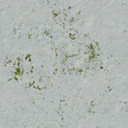 |
ceylon\ground_sand4_ceylon |
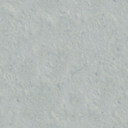 |
ceylon\ground_shoreline1_ceylon |
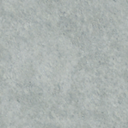 |
ceylon\ground_shoreline2_ceylon |
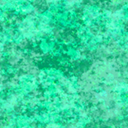 |
ceylon\seafloor0_ceylon |
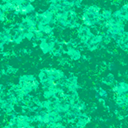 |
ceylon\seafloor1_ceylon |
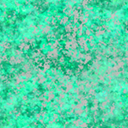 |
ceylon\seafloor2_ceylon |
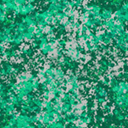 |
ceylon\seafloor3_ceylon |
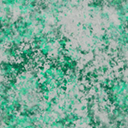 |
ceylon\seafloor4_ceylon |
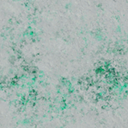 |
ceylon\seafloor5_ceylon |
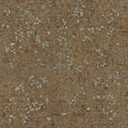 |
city\ground1_cob |
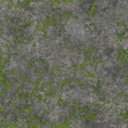 |
coastal_japan\ground_dirt1_co_japan |
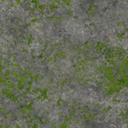 |
coastal_japan\ground_dirt2_co_japan |
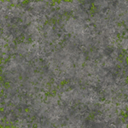 |
coastal_japan\ground_dirt3_co_japan |
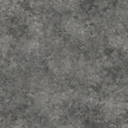 |
coastal_japan\ground_dirt4_co_japan |
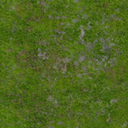 |
coastal_japan\ground_forest_co_japan |
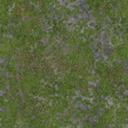 |
coastal_japan\ground_grass1_co_japan |
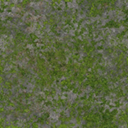 |
coastal_japan\ground_grass2_co_japan |
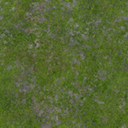 |
coastal_japan\ground_grass3_co_japan |
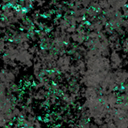 |
coastal_japan\seafloor_coral1_co_japan |
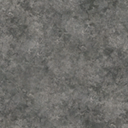 |
coastal_japan\shoreline1_co_japan |
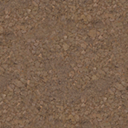 |
deccan\ground_dirt2_deccan |
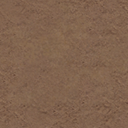 |
deccan\ground_dirt3_deccan |
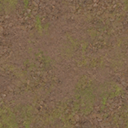 |
deccan\ground_dirt4_deccan |
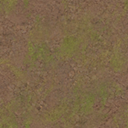 |
deccan\ground_dirt5_deccan |
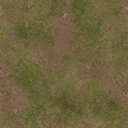 |
deccan\ground_forest_deccan |
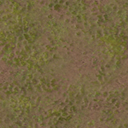 |
deccan\ground_grass1_deccan |
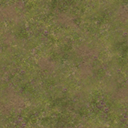 |
deccan\ground_grass2_deccan |
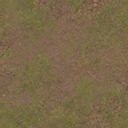 |
deccan\ground_grass3_deccan |
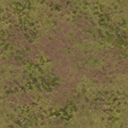 |
deccan\ground_grass4_deccan |
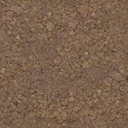 |
deccan\wall_deccan |
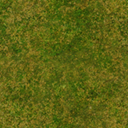 |
great_lakes\ground_grass1_gl |
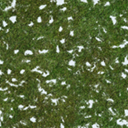 |
great_lakes\ground_grass2_gl |
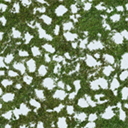 |
great_lakes\ground_grass3_gl |
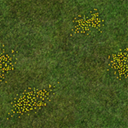 |
great_lakes\ground_grass4_gl |
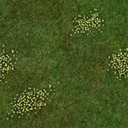 |
great_lakes\ground_grass5_gl |
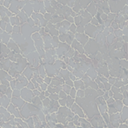 |
great_lakes\ground_ice1_gl |
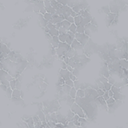 |
great_lakes\ground_ice2_gl |
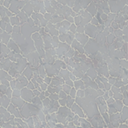 |
great_lakes\ground_ice3_gl |
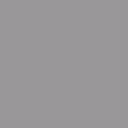 |
great_lakes\ground_shoreline1_gl |
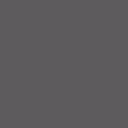 |
great_lakes\ground_shoreline2_gl |
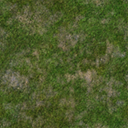 |
great_lakes\ground_shoreline3_gl |
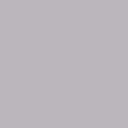 |
great_lakes\ground_snow1_gl |
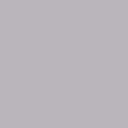 |
great_lakes\ground_snow2_gl |
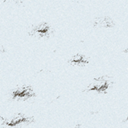 |
great_lakes\ground_snow3_gl |
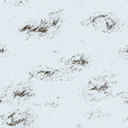 |
great_lakes\ground_snow4_gl |
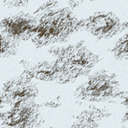 |
great_lakes\ground_snow5_gl |
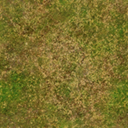 |
great_lakes\groundforest_gl |
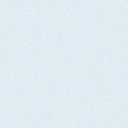 |
great_lakes\groundforest_snow_gl |
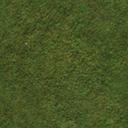 |
great_plains\default |
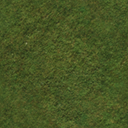 |
great_plains\ground1_gp |
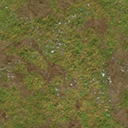 |
great_plains\ground2_gp |
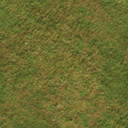 |
great_plains\ground3_gp |
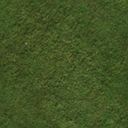 |
great_plains\ground4_gp |
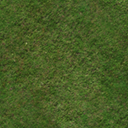 |
great_plains\ground5_gp |
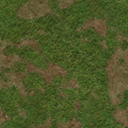 |
great_plains\ground6_gp |
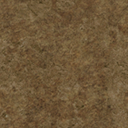 |
great_plains\ground7_gp |
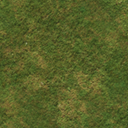 |
great_plains\ground8_gp |
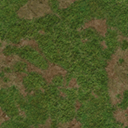 |
great_plains\groundforest_gp |
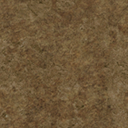 |
great_plains\groundSPC_nonpass_gp |
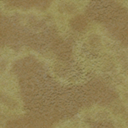 |
great_plains\pondbank_gp |
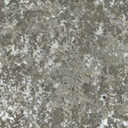 |
himalayas\cliff_himal_edge |
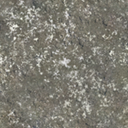 |
himalayas\cliff_himal_top |
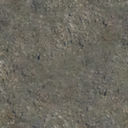 |
himalayas\ground_dirt1_himal |
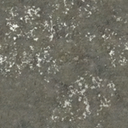 |
himalayas\ground_dirt2_himal |
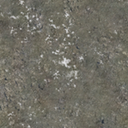 |
himalayas\ground_dirt3_himal |
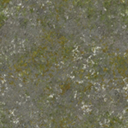 |
himalayas\ground_dirt4_himal |
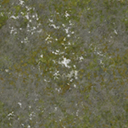 |
himalayas\ground_dirt5_himal |
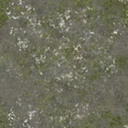 |
himalayas\ground_dirt6_himal |
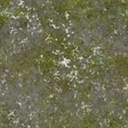 |
himalayas\ground_dirt7_himal |
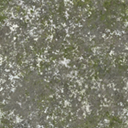 |
himalayas\ground_dirt8_himal |
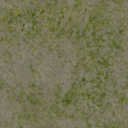 |
mongolia\ground_forest_mongol |
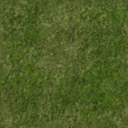 |
mongolia\ground_grass1_mongol |
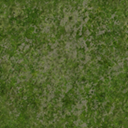 |
mongolia\ground_grass2_mongol |
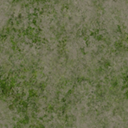 |
mongolia\ground_grass3_mongol |
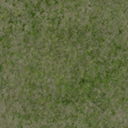 |
mongolia\ground_grass4_mongol |
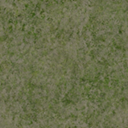 |
mongolia\ground_grass5_mongol |
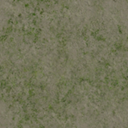 |
mongolia\ground_grass6_mongol |
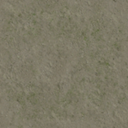 |
mongolia\ground_sand1_mongol |
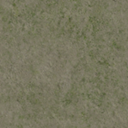 |
mongolia\ground_sand2_mongol |
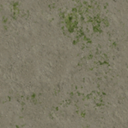 |
mongolia\ground_sand3_mongol |
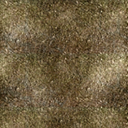 |
new_england\cliff_edge_ne |
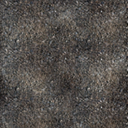 |
new_england\cliff_inland_edge_ne |
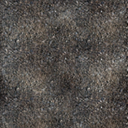 |
new_england\cliff_inland_side_ne |
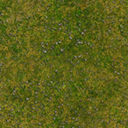 |
new_england\cliff_inland_top_ne |
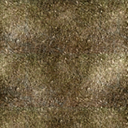 |
new_england\cliff_side_ne |
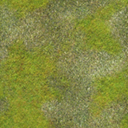 |
new_england\cliff_top_ne |
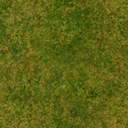 |
new_england\ground1_ne |
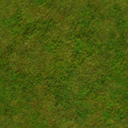 |
new_england\ground2_cliff_ne |
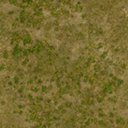 |
new_england\ground2_ne |
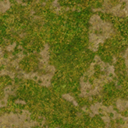 |
new_england\ground3_ne |
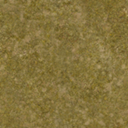 |
new_england\ground4_ne |
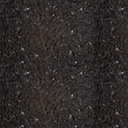 |
new_england\ground5_ne |
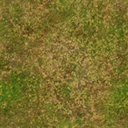 |
new_england\groundforest_ne |
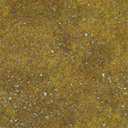 |
new_england\river1_ne |
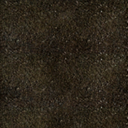 |
new_england\shoreline1_ne |
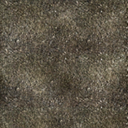 |
new_england\shoreline2_ne |
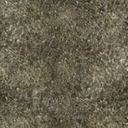 |
new_england\shoreline3_ne |
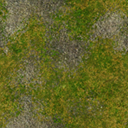 |
new_england\shoreline4_ne |
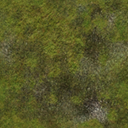 |
new_england\shoreline5_ne |
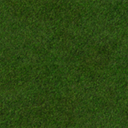 |
nwterritory\ground_grass1_nwt |
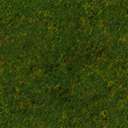 |
nwterritory\ground_grass1a_nwt |
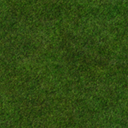 |
nwterritory\ground_grass2_nwt |
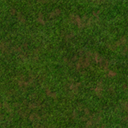 |
nwterritory\ground_grass3_nwt |
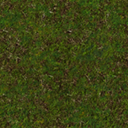 |
nwterritory\ground_grass5_nwt |
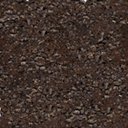 |
nwterritory\ground_riverbed_nwt |
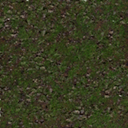 |
nwterritory\ground_riverbed2_nwt |
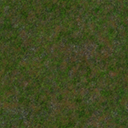 |
nwterritory\ground_shoreline3_nwt |
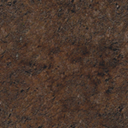 |
nwterritory\ground_shoreline4_nwt |
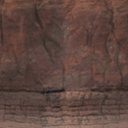 |
Painteddesert\pd_cliff_diffuse_a |
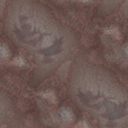 |
Painteddesert\pd_ground_diffuse_a |
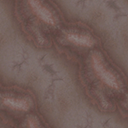 |
Painteddesert\pd_ground_diffuse_b |
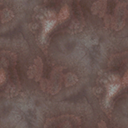 |
Painteddesert\pd_ground_diffuse_c |
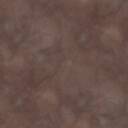 |
Painteddesert\pd_ground_diffuse_d |
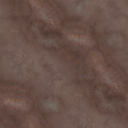 |
Painteddesert\pd_ground_diffuse_e |
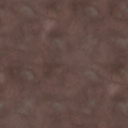 |
Painteddesert\pd_ground_diffuse_f |
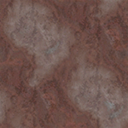 |
Painteddesert\pd_ground_diffuse_g |
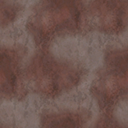 |
Painteddesert\pd_ground_diffuse_h |
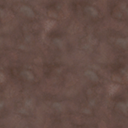 |
Painteddesert\pd_ground_diffuse_i |
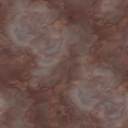 |
Painteddesert\pd_ground_diffuse_j |
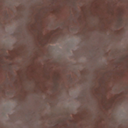 |
Painteddesert\pd_ground_diffuse_k |
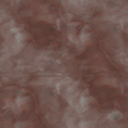 |
Painteddesert\pd_ground_diffuse_l |
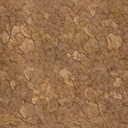 |
pampas\ground1_pam |
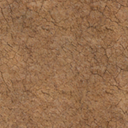 |
pampas\ground2_pam |
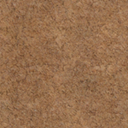 |
pampas\ground3_pam |
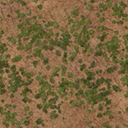 |
pampas\ground4_pam |
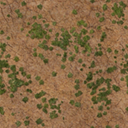 |
pampas\ground5_pam |
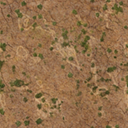 |
pampas\ground6_pam |
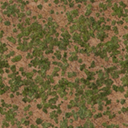 |
pampas\groundforest_pam |
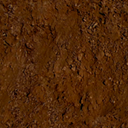 |
pampas\river_shoreline1_pam |
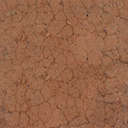 |
pampas\river_shoreline2_pam |
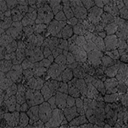 |
pampas\river_shoreline3_pam |
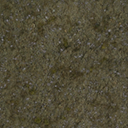 |
patagonia\ground_clifftop_pat |
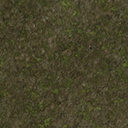 |
patagonia\ground_dirt1_pat |
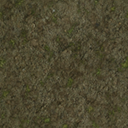 |
patagonia\ground_dirt2_pat |
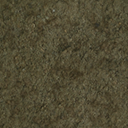 |
patagonia\ground_dirt3_pat |
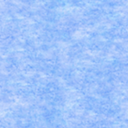 |
patagonia\ground_glacier_pat |
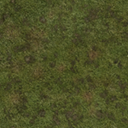 |
patagonia\ground_grass1_pat |
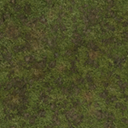 |
patagonia\ground_grass2_pat |
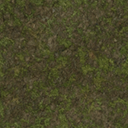 |
patagonia\ground_grass3_pat |
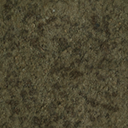 |
patagonia\ground_shoreline1_pat |
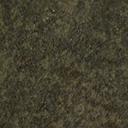 |
patagonia\ground_shoreline2_pat |
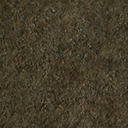 |
patagonia\ground_shoreline3_pat |
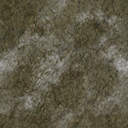 |
patagonia\ground_snow1_pat |
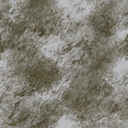 |
patagonia\ground_snow2_pat |
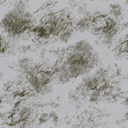 |
patagonia\ground_snow3_pat |
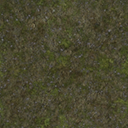 |
patagonia\groundforest_pat |
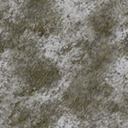 |
patagonia\groundforest_snow_pat |
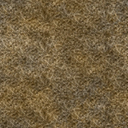 |
props\east_colony |
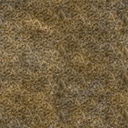 |
props\east_colony1 |
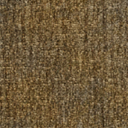 |
props\east_colony2 |
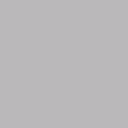 |
rockies\clifftop_roc |
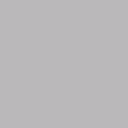 |
rockies\clifftop_roc |
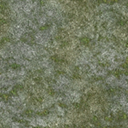 |
rockies\ground1_roc |
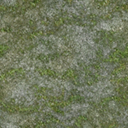 |
rockies\ground2_roc |
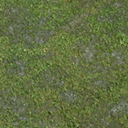 |
rockies\ground3_roc |
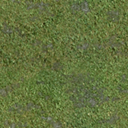 |
rockies\ground4_roc |
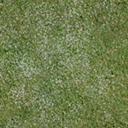 |
rockies\ground5_roc |
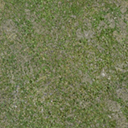 |
rockies\groundforest_roc |
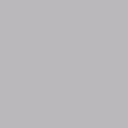 |
rockies\groundforestsnow_roc |
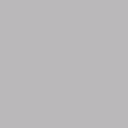 |
rockies\groundsnow1_roc |
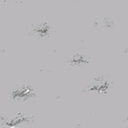 |
rockies\groundsnow2_roc |
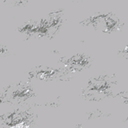 |
rockies\groundsnow3_roc |
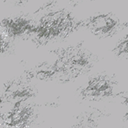 |
rockies\groundsnow4_roc |
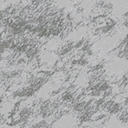 |
rockies\groundsnow5_roc |
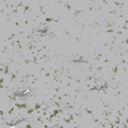 |
rockies\groundsnow6_roc |
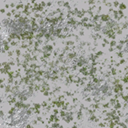 |
rockies\groundsnow7_roc |
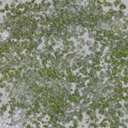 |
rockies\groundsnow8_roc |
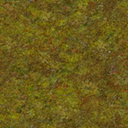 |
Saguenay\ground1_sag |
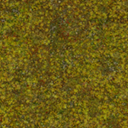 |
Saguenay\ground2_sag |
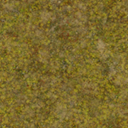 |
Saguenay\ground3_sag |
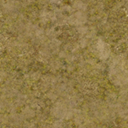 |
Saguenay\ground4_sag |
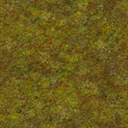 |
Saguenay\ground5_sag |
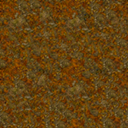 |
Saguenay\ground6_sag |
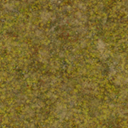 |
saguenay\groundforest_sag |
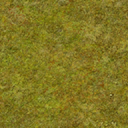 |
saguenay\river1_sag |
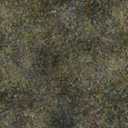 |
saguenay\shoreline1_sag |
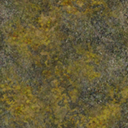 |
saguenay\shoreline2_sag |
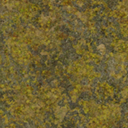 |
saguenay\shoreline3_sag |
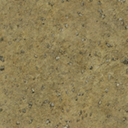 |
sonora\cliff_edge_son |
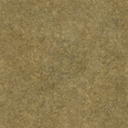 |
sonora\ground1_son |
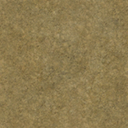 |
sonora\ground1clifftop_son |
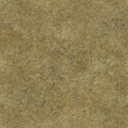 |
sonora\ground2_son |
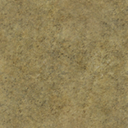 |
sonora\ground3_son |
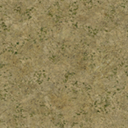 |
sonora\ground4_son |
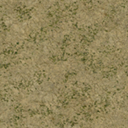 |
sonora\ground5_son |
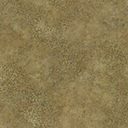 |
sonora\ground6_son |
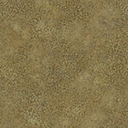 |
sonora\ground7_son |
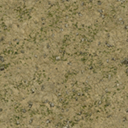 |
sonora\groundforest_son |
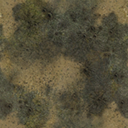 |
spc\scorched01 |
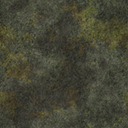 |
spc\scorched02 |
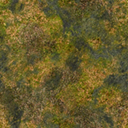 |
spc\scorched03 |
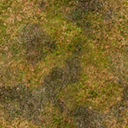 |
spc\scorched04 |
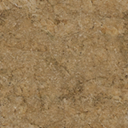 |
Texas\cliff_edge_tex |
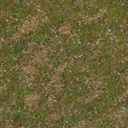 |
Texas\cliff_top_grass_tex |
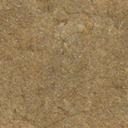 |
Texas\cliff_top_tex |
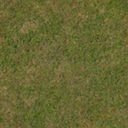 |
Texas\ground1_tex |
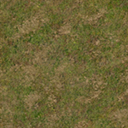 |
Texas\ground2_tex |
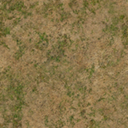 |
Texas\ground3_tex |
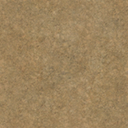 |
Texas\ground4_tex |
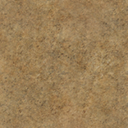 |
Texas\ground5_tex |
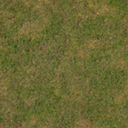 |
Texas\ground6_tex |
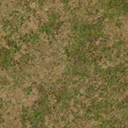 |
texas\groundforest_tex |
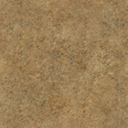 |
texas\groundforestdirt_tex |
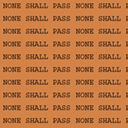 |
texas\nonpassable_temp |
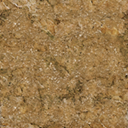 |
texas\river1_tex |
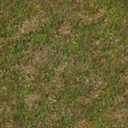 |
Texas\river2_tex |
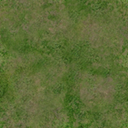 |
Yellow_river\forest_yellow_riv |
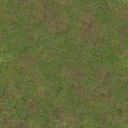 |
Yellow_river\grass1_yellow_riv |
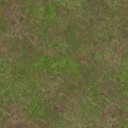 |
Yellow_river\grass2_yellow_riv |
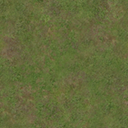 |
Yellow_river\grass3_yellow_riv |
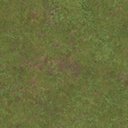 |
Yellow_river\grass4_yellow_riv |
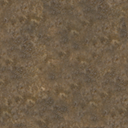 |
Yellow_river\shoreline1_yellow_riv |
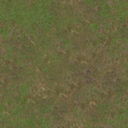 |
Yellow_river\stone1_yellow_riv |
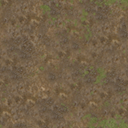 |
Yellow_river\stone2_yellow_riv |
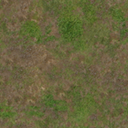 |
Yellow_river\stone3_yellow_riv |
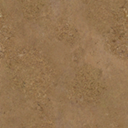 |
yucatan\ground_shoreline1_yuc |
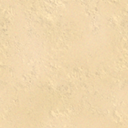 |
yucatan\ground_shoreline2_yuc |
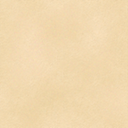 |
yucatan\ground_shoreline3_yuc |
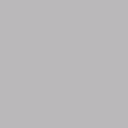 |
yukon\ground1_yuk |
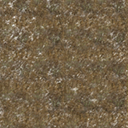 |
yukon\ground10_yuk |
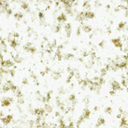 |
yukon\ground11_yuk |
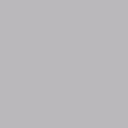 |
yukon\ground2_yuk |
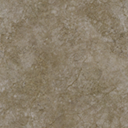 |
yukon\ground3_SPCnonbuild_yuk |
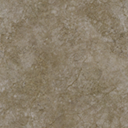 |
yukon\ground3_yuk |
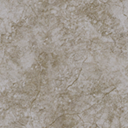 |
yukon\ground3x_yuk |
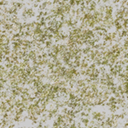 |
yukon\ground4_yuk |
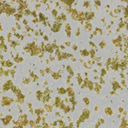 |
yukon\ground5_yuk |
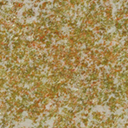 |
yukon\ground6_yuk |
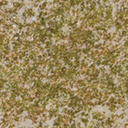 |
yukon\ground7_yuk |
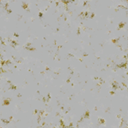 |
yukon\ground8_yuk |
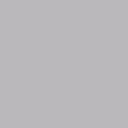 |
yukon\ground9_yuk |
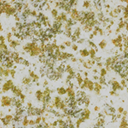 |
yukon\groundforest_yuk |
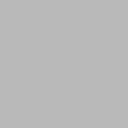 |
yukon\groundforestsnow_yuk |